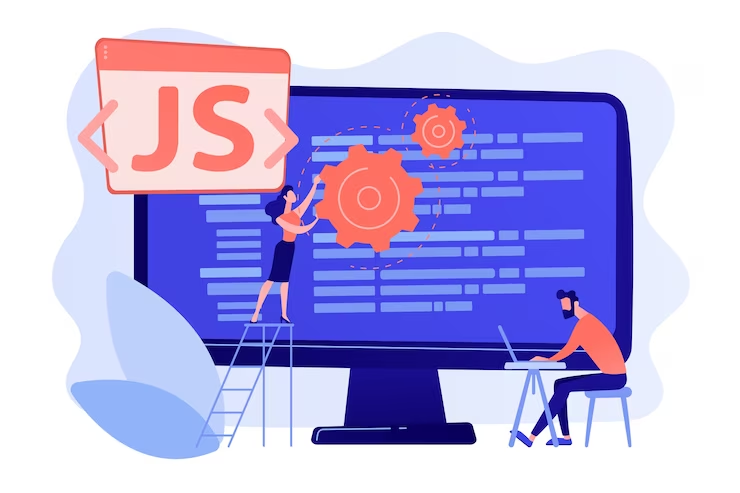
JavaScript Remove Element: Web Page Manipulation in 2023
In the ever-evolving world of web development, staying updated with the latest JavaScript techniques is essential. In this guide, we will not only walk you through the process of removing an element from a web page using the JavaScript Remove Method but also provide you with the most current information for the year 2023.
Whether you’re a seasoned developer or just starting your coding journey, this comprehensive tutorial will equip you with the knowledge you need to master this essential skill.
Understanding the JavaScript Remove Method
The JavaScript Remove Method is a powerful tool that allows you to remove elements from your HTML document dynamically. It’s a straightforward and efficient way to manipulate the content of your web page. Let’s dive into the step-by-step process of using this method.
Selecting the Element to Remove
First, you need to select the element you want to remove. You can do this by using various methods like `getElementById`, `querySelector`, or `getElementsByClassName`. For example:
const elementToRemove = document.getElementById('your-element-id');
Removing the Element
Once you have selected the element, removing it is as simple as one line of code:
elementToRemove.remove();
This line tells the browser to remove the selected element from the DOM (Document Object Model), effectively erasing it from your web page.
The 2023 Update: Modern Best Practices
In 2023, web development has advanced, and best practices have evolved. Here are some additional tips to keep in mind.
Check for Element Existence
Before removing an element, it’s a good practice to check if it exists. This prevents potential errors in case the element is not present on the page. You can use an `if` statement to ensure its existence:
if (elementToRemove) {
elementToRemove.remove();
} else {
console.log('Element does not exist.');
}
Use Event Delegation
In modern web development, event delegation is preferred. Instead of removing elements directly, you can add event listeners to a parent element and remove the target element when an event is triggered. This is particularly useful for dynamic content:
const parentElement = document.getElementById('parent-element-id');
parentElement.addEventListener('click', function (event) {
if (event.target.classList.contains('remove-button')) {
event.target.parentElement.remove();
}
});
Conclusion
Mastering the JavaScript Remove Method is an essential skill for any web developer in 2023. This guide has provided you with a comprehensive overview of how to use this method effectively. Remember to stay up-to-date with modern best practices, including checking for element existence and utilizing event delegation.
With these techniques, you can confidently manipulate your web page’s content and create dynamic and responsive user interfaces. As the world of web development continues to evolve, your knowledge of JavaScript will be your key to success.